Pattern Sections
Pattern: Parameter Forest
also known as: Parameter Comb, Hybrid Parameter List
Context
An API provider wants to offer one or more Operations to API clients using an API endpoint. To enable the communication, they need to agree on the structure of each message (i.e, the request message and response message of the Operation) to be exchanged.
Problem
How can multiple Parameter Trees be exposed as request or response payload of an API operation?
Forces
The data structures of the request messages and response messages are an essential part of the API contract. The following forces (shared with Atomic Parameter, Atomic Parameter List, and Parameter Tree) need to be balanced:
- Structure of the domain model and the system behavior and its impact on understandability (including considerations of simplicity, complexity, and traceability)
- Additional data to be transmitted (such as security-related data or metadata)
- Performance (latency, message processing) and resource use (bandwidth, memory, CPU power)
- Loose coupling and interoperability
- Developer convenience and experience
- Security and data privacy
Pattern forces are explained in depth in the book.
Solution
Define a Parameter Forest comprising two or more Parameter Trees. Locate the forest members by position or name.
Sketch
A solution sketch for this pattern from pre-book times is:
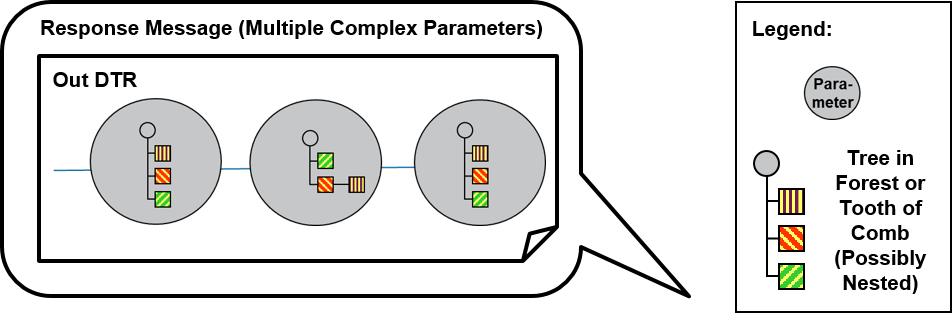
Example
The following interface demonstrates all four basic representation
patterns using alias names for the four patterns: Dot
(for
Atomic
Parameter), Dotted Line
(for
Atomic
Parameter List), Bar
(for
Parameter
Tree), and Comb
(for Parameter Forest). That
is, it shows how a Parameter Forest can be build up based on
the more simple patterns. Here is the corresponding Java code:
@WebService
public interface IRPService {
boolean dotInDotOut(int singleScalarParameter);
int dottedLineInDotOut(String scalarParameter1, String scalarParameter2);
ResponseDTO barInBarOut(RequestDTO singleComplexParameter);
ResponseDTO combInBarOut(RequestDTO complexParameter1,
AnotherRequestDTO complexParameter2);
}
public class RequestDTO {
private float value;
private String unit;
[...]
}
public class AnotherRequestDTO {
private int id;
private NestedRequestDTO[] toothOfComb;
[...]
public class ResponseDTO {
private int id;
private String dataField1;
private String dataField2;
[...]
}
Here are the corresponding XSD types:
<xs:complexType name="combInBarOut">
<xs:sequence>
<xs:element minOccurs="0" name="arg0" type="tns:requestDTO"/>
<xs:element minOccurs="0" name="arg1" type="tns:anotherRequestDTO"/>
</xs:sequence>
</xs:complexType>
<xs:complexType name="requestDTO">
<xs:sequence>
<xs:element minOccurs="0" name="unit" type="xs:string"/>
<xs:element name="value" type="xs:float"/>
</xs:sequence>
</xs:complexType>
<xs:complexType name="anotherRequestDTO">
<xs:sequence>
<xs:element name="id" type="xs:int"/>
<xs:element maxOccurs="unbounded" minOccurs="0"
name="toothOfComb" nillable="true" type="tns:nestedRequestDTO"/>
</xs:sequence>
</xs:complexType>
<xs:complexType name="nestedRequestDTO">
<xs:sequence>
<xs:element minOccurs="0" name="key" type="xs:string"/>
<xs:element minOccurs="0" name="valie" type="xs:string"/>
</xs:sequence>
</xs:complexType>
<xs:complexType name="responseDTO">
<xs:sequence>
<xs:element minOccurs="0" name="dataField1" type="xs:string"/>
<xs:element minOccurs="0" name="dataField2" type="xs:string"/>
<xs:element name="id" type="xs:int"/>
</xs:sequence>
</xs:complexType>
The combInBarOut
request message has two parameters each
forming one “tooth” of the “comb” (in the metaphor); both of these
parameters are further structured into Data Transfer Objects
(DTOs).
Are you missing implementation hints? Our papers publications provide them (for selected patterns).
Consequences
The resolution of pattern forces and other consequences are discussed in our book.
Known Uses
The response messages of the core banking integration solution described in Brandner et al. (2004) use this pattern by providing domain-specific complex types in XML Schema (XSD).
The Flickr App
Garden uses Parameter Forests in multiple calls, e.g., in
the responses of its read access to collections: flickr.collections.getInfo
.
Note that the Flickr API and App Garden also use the
Atomic
Parameter List pattern, e.g. for Upload Photos
.
The API supports multiple message exchange formats in its request and
response messages, including SOAP, RESTful mime/media types, and even
XML-RPC.
The response structure used in the Twitter REST API (https://dev.twitter.com/rest/collections/responses) also uses a Parameter Forest, with one tree containing an array of objects and a second one containing control information and metadata such as cursors and page tokens (see Pagination pattern).
A message created with the Protocol
Buffers data interchange format, originally developed by Google and
open sourced at GitHub,
that contains another message that is tagged with the keyword
repeated
uses this pattern.
JSON API Message
Responses are instances of this pattern, with three mandatory
members (data
, errors
, meta
) and
three optional ones (jsonapi
, links
,
included
). Each one of those is a
Parameter
Tree.
More Information
Related Patterns
As an alternative to a Parameter Forest multiple messages using the simpler structures Atomic Parameter, Atomic Parameter List, or Parameter Tree are possible. When it makes sense, the elements you would structure as a Parameter Forest can also be structured in a Parameter Tree, all under a single root element; if the data elements are rather unrelated, like the content and metadata examples in the known uses (e.g., Twitter REST API), Parameter Forest is better suited as it more clearly reflects the intent of the structure.
Parameter Forest uses Atomic Parameters, Atomic Parameter Lists, or Parameter Trees and even other nested Parameter Forests as its building blocks, as explained above.
Like Atomic Parameter, Atomic Parameter List and Parameter Tree, this pattern can be used in the three patterns Command Message, Document Message, and Event Message from Hohpe and Woolf (2003), if the content can be represented as a number of complex structures. For instance, a command, event or document described as Parameter Tree can be actually sent as a Parameter Forest if additional information need to be included, such as security metadata or other metadata.
Other Sources
As described in Hohpe and Woolf (2003), often messages originating from external systems contain many levels of nested, repeating data elements as they are modeled after generic, normalized database structures. The resulting message structure is than often a Parameter Tree or Parameter Forest. Content Filters Hohpe and Woolf (2003) can be used to simplify such structures, e.g., into a list, if not all the information is needed. Some Content Enrichers Hohpe and Woolf (2003) in turn retrieve data from such external systems and enrich messages with such data; hence, a Parameter Tree or a Parameter Forest is often the resulting data structure.